Since Flutter’s launch in Dec 2018, many teams have made their hands dirty with Flutter development. Industry leaders like Google, Alibaba have amazed us with their masterpieces built using the Flutter app development. To get started, you can:
Check out our in-depth Flutter Tutorial for Beginners
One of the dilemmas that developers (or CTOs) might have is if it would be possible to integrate existing third-party Native SDKs (and functionality) into Flutter apps. Good news — It is very much possible.
Assume that you want to integrate a video chat platform such as Tokbox’s SDK (for the Video chat / messaging functionality) into your Flutter app. As of now, Tokbox doesn’t have any native SDK (library) for Flutter. Therefore to use the functionality, there are two ways to go about this:
Flutter Native Third-Party SDK-UI integration
You can directly call native Android and iOS SDKs of Tokbox chat app from your Flutter application — wherein the UI will be driven by Tokbox SDKs. Since this approach uses native UIs of each platform, the app developer is devoid of any responsibility to create working UIs on both the Android and iOS platforms.
A downside of this approach is that even if the whole app supports say iOS 9 (because it’s Flutter), because of one SDK — you will have to support the minimum OS version that all Flutter third party SDKs support!
On the flip side, your development time (including Project Management and QA) and thus costs are reduced.
Dart UI + Flutter Native SDK
Create a wrapper around the Flutter native SDKs and create a separate UI (built-in dart) that will be used across both the iOS and Android versions — assuming that the Flutter native SDKs will expose the necessary methods.
Going with this approach is that you will have to invest significant time and thus resources to create a Dart UI (Design + Development) which will ultimately offer much better control. Thus you will still be able to support that minimum OS as well as offer a better User Experience.
The Concept
Before we dig into the “how”, let’s dig into the “why”. Let’s understand what are Flutter Platform channels. Flutter uses a flexible system that allows you to write a platform-specific code either in Java or in Kotlin for Android & in Swift or Objective-C for iOS.
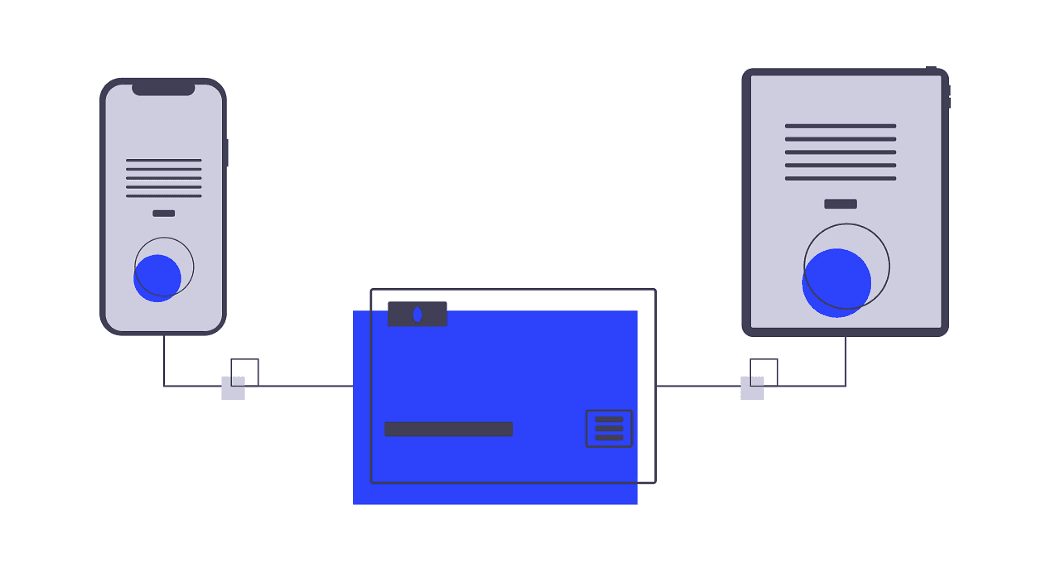
It works on the below message-passing style:
Step1: Flutter portion of the app sends a message to its host app (Android or iOS portion of Application)
Step 2: The host listens to the Platform channels and receives the message.
Step 3: Host apps execute the Flutter Native code (written in Platform-specific language)
Step 4: Host apps return the response to the Flutter portion of the app.
The below diagram illustrates the architecture of the Platform channel in Flutter.
Example of Tokbox Flutter Video Chat SDK Integration
Now let’s integrate the Video chat feature of https://www.vonage.com/communications-apis/video/ to our Flutter application and understand this step by step. I’ll be following the Native SDK-UI integration (first) approach to demonstrate the Platform channel.
I assume you have already created a Flutter application using
flutter create my_video_call_demo
Now go to main.dart and follow the below steps.
Step 1: Constructing Method channel
First, we will construct a method channel which will allow us to communicate between Platform-specific code and Flutter code.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
class _HomeWidgetState extends State<HomeWidget> {
static const platform = const MethodChannel("com.stl.flutchat/opentok");
//Invoke mthod via method channel instance
}
Step 2: Invoke platform Specific method from Flutter
Next, we can invoke any method using this method channel. This method may fail if the target platform doesn’t support the Platform API or throws an Exception. So here PlatformException should be handled well.
String _callDuration;
void _openVideoCallScreen() async {
String callDuration = "Unknown call duration.";
try {
callDuration = await platform.invokeMethod("openVideoChat");
} on PlatformException catch (_) {
callDuration = "Failed to get call duration.";
}
setState(() {
_callDuration = callDuration;
});
}
Step 3.1: Add Android-specific Implementation
Now Android portion of the host app will listen to the Platform channel and receives a message to invoke a method.
We will integrate Tokbox related code using Android SDKs in a separate Activity called VideoScreen. (Full source code is available at the end of the article)
We will start the respective activity when a particular method is invoked via method channel.
import android.os.Bundle
import io.flutter.app.FlutterActivity
import io.flutter.plugin.common.MethodChannel
import io.flutter.plugins.GeneratedPluginRegistrant
class FirstActivity : FlutterActivity() {
private var result: MethodChannel.Result? = null
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
GeneratedPluginRegistrant.registerWith(this)
MethodChannel(flutterView, "com.stl.flutchat/opentok")
.setMethodCallHandler { methodCall, result ->
methodCall.method?.let {
if (it.contentEquals("openVideoChat")) {
this@FirstActivity.result = result
startActivityForResult(Intent( this, VideoActivity::class.java), 300)
}
}
}
}
}
Step 3.2: Returning result from Native to Flutter
MethodCallHandler gives access to MethodCall and MethodChannel.Result. The result allows us to pass data back to the Flutter portion of the app using Success, Error, and notImplemented() method.
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == 300) {
data?.extras?.let { it ->
val callStartDate: Date = it.getSerializable("callStartTime") as Date
result?.success("${callStartDate.getDuration().first}:" +
"${callStartDate.getDuration().second}:" +
callStartDate.getDuration().third)
}
}
}
Step 4: Add iOS-specific Implementation
Similar to Android, in the iOS host application, we will integrate Tokbox Flutter native iOS SDKs and present VideChatScreen when a particular method is invoked via method channel.
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?
) -> Bool {
let controller : FlutterViewController = window?.rootViewController as! FlutterViewController
let videoChatChannel = FlutterMethodChannel(name: "com.stl.flutchat/opentok",
binaryMessenger: controller)
videoChatChannel.setMethodCallHandler({
[weak self] (call: FlutterMethodCall, result: @escaping FlutterResult) -> Void in
switch call.method {
case "openVideoChat":
self?.presentVideoChatScreen(result: result)
default:
result(FlutterMethodNotImplemented)
}
})
GeneratedPluginRegistrant.register(with: self)
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
All Good!, Now we are able to work with native SDKs to our Flutter app. The full source code of an application can be found from the below URL.
With the team gets ready, Challenges don’t stop there! It is equally challenging to prove to your Clients/Product owners that you can start using Flutter for production apps. If they are still evaluating Flutter, here is everything that you will need to change their minds :)
And if you’re looking for more proof of how much Flutter is already being adopted by companies around the world, check out the showcase.